Import assets
To understand how assets are imported into Manim, you first have to set a workspace, that is, a folder.
It is advisable to create a folder for assets and each type of document, for example:
.
├── assets
│ ├── images
│ ├── sounds
│ └── svg
│
└── your_script.py
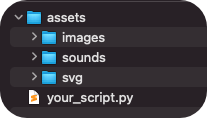
Images and SVG
Manim supports PNG images (opaque or with transparency), JPEG, JPG and SVG. However, SVGs must be well built, that is, they must not have errors in their code for it to work.
Download the following files:
Normal raster image:
test_image
.PNG with transparency:
test_transparency
.SVG:
svg_test
.
And locate them as follows:
├── assets
│ ├── images
│ │ ├── test_image.png
│ │ └── test_transparency.png
│ │
│ └── svg
│ └── svg_test.svg
│
└── your_script.py
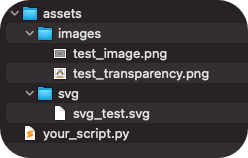
The way to import it is as follows:
def construct(self):
# Change background color only this scene
self.camera.background_color = TEAL
test_image = ImageMobject("assets/images/test_image.png")
test_image.to_corner(LEFT)
test_image.set(width=3)
test_transparency = ImageMobject("assets/images/test_transparency.png")
test_transparency.set(width=3)
svg = SVGMobject("assets/svg/svg_test")
svg.set(width=3)
svg.to_edge(RIGHT)
self.add(
test_image,
test_transparency,
svg
)
self.wait()
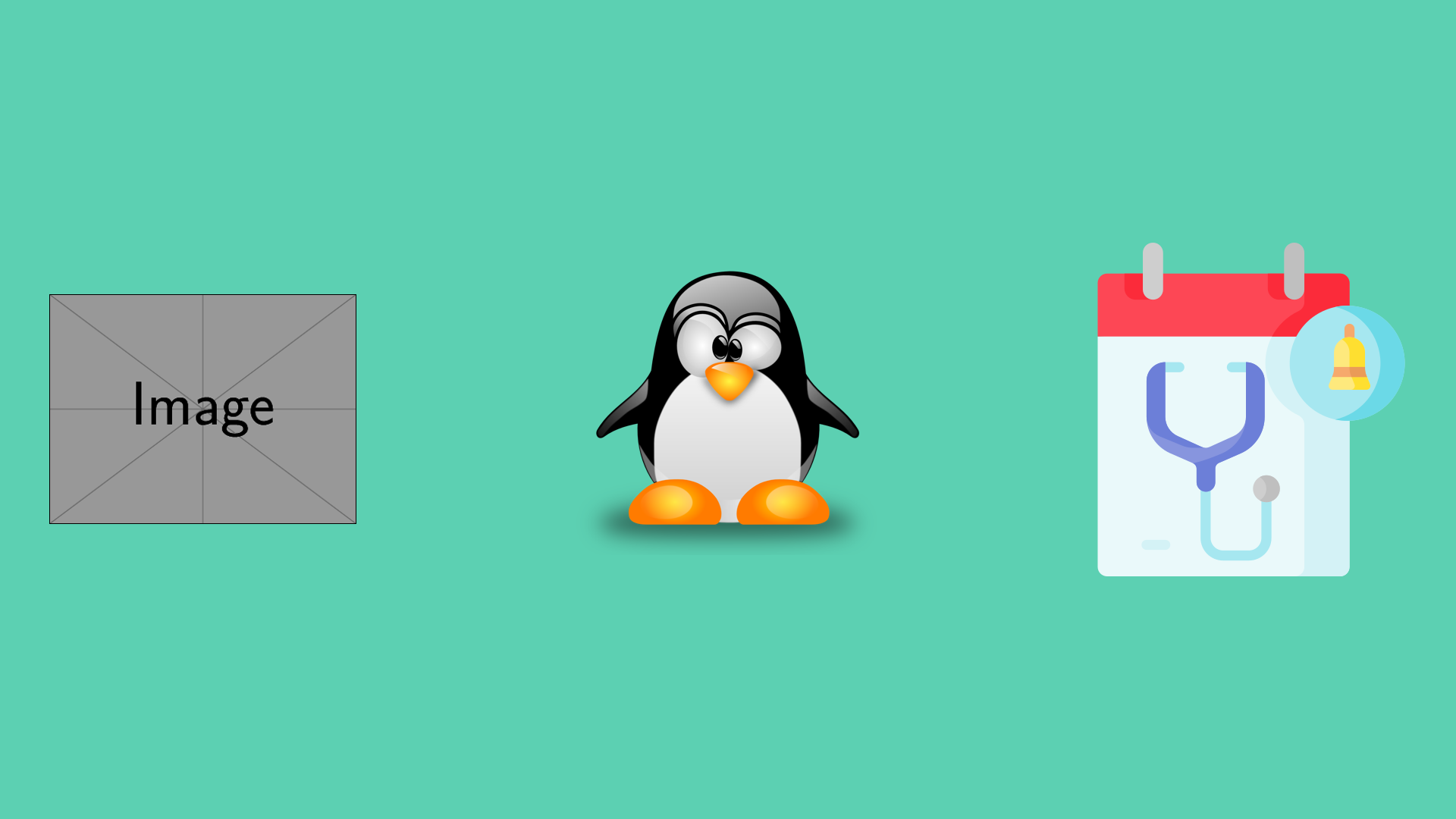
Note
Note that ImageMobject
and SVGMobject
refer to the directory where you are running Manim (your workspace).
Warning
Class animations that were designed for VMobjects
cannot be applied to ImageMobjects
. ImageMobjects
are not VMobjects
. For example, Write
cannot be applied to an ImageMobject
, but FadeIn
or FadeOut
can.
Sounds
The same logic is applied as the images, it is recommended to create a folder called sounds
, here is an example, download the following files and locate them in this way:
├── assets
│ └── sounds
│ ├── count.wav
│ └── finish.wav
│
└── your_script.py
Sound 1:
count.wav
.Sound 2:
finish.wav
.
Code:
def construct(self):
for i in range(5):
t = Text(f"{i+1}")
t.set(height=config.frame_height - 2)
self.add(t)
if i != 4:
# "gain" is the amplification of the sound
self.add_sound("assets/sounds/count.wav",gain=3)
else:
self.add_sound("assets/sounds/finish.wav",gain=3)
self.wait()
self.remove(t)
Warning
It is recommended to use the --flush_cache
flag, as sometimes the cache can cause the rendering to not work quite well, example:
manim your_script.py SomeScene -pqm --flush_cache